Switch Documentation
- Whitelist your IP(s) or your messages and download requests will be rejected. Do this on the dashboard: MANAGE ACCOUNT -> MANAGE IPS AND ENDPOINTS.
- Find your MD5 hashed version of your password. When your system was registered on WENO’s cert dashboard you received your API Partner ID and password. You must use the MD5 hashed version of your API Partner password in the headers of your Rx messages. The MD5 hashed version can found in your Account Details page under the “Company Data” section.
This step will auto complete after you download, by computer call, at least once, each file you have access to in your ACCOUNT DETAILS page.
We provide sample code below for you to download the files you have access to. If you do not do full file replacements as recommended here YOU WILL HAVE ERRORS using wrong pharmacy or drug data in your application.
- Pharmacy – replace the full file at least weekly
- DrugDB – update after 25th of each month.
TIP: How to use a drug’s ingredient list for allergy alerts
TIP: How to work with drug to drug interaction data
Your list of test pharmacies are shown here and flagged as TEST pharmacies in your Pharmacy Directory. Do not send tests NewRxs to real pharmacies without WENO’s permission, which will not be unreasonably withheld.
Pharmacy Name | NCPDP |
Test Direct Pharmacy | 1234567 |
Test WOL Registered Pharmacy | 7654321 |
Test WOL Pending Pharmacy | TestWOLPendingPharmacy |
Test WOL Fax Alert Failure Pharmacy | TestWOLFaxAlertFailurePharmacy |
Test Transmission Failure | TestTransmissionFailure |
Test ERx Rejected By Pharmacy | TestERxRejectedByPharmacy |
Use the NCPDP value in the TO field of the Header with “P” as qualifier, or “ZZZ” as the qualifier for international pharmacy testing.
When a Pharmacy is not in our Pharmacy Directory
International pharmacies must be found in our directory (contact us to add), but you can route NewRx to any US pharmacy even if it is not found in our directory by following the following steps:
Step 1: TO field of Header needs “P” qualifier with the pharmacy 7 digits NCPDP ID in value. Step 2: Pharmacy segment of NewRx needs:
– NPI value populated
– Business name populated
– Address Line 1, City, State, Zip populated
– Primary Phone populated
– Fax populated
Prioritize Pharmacies for best results
If a pharmacy connectivity status on WENO Pharmacy Directory is listed as “registered” or “direct”, a GOOD practice would be to prioritize these pharmacies to prescribers. For ex. make all pharmacies available to ePrescribe to but when they find/select them on your screen, emphasize these somehow (a green light or top of the list, or sortable by those already connected to WENO, WENO lovin, WENO friendly, etc.).
Manual Downloads
Go to your Manage Account navigation bar to manually download the pharmacy directory from the Manage List page or the the drug database from the Drug Database page. If you purchased the drug database and do not see how to access it, contact us.
About the Drug Database
- This is a Sample Drug Database excel file that explains the drug database file that you will access:
- Sheet 1 is a sample of the file which is returned in a format which can be consumed by your system.
- Sheet 2 is an excel spreadsheet of same which maps to sheet 3.
- Sheet 3 explains how to use the data for your user interface and ePrescribing transactions.
Sample Code to Access Files You Have Permission For:
PHP Code
// weno_get_file.php version 1.2
// run from console: php weno_get_file.php
$dir = ['DrugDBDirectory', 'PharmacyDirectory', 'PrescriberDirectory'];
$choice = 0; // 0 drugs, 1 pharmacies, 2 prescribers
$localfilename = "{$dir[$choice]}.zip";
// for live data use live.wenoexchange.com instead
$url = "https://cert.wenoexchange.com/wenox/GetListResponse.aspx?{$dir[$choice]}=yes";
// provide your Partner ID and password MD5 hash
// as shown on your dashboard under Account details -> Company Data
$partnerid = 'xxx';
$pass_hash = 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx';
$out = fopen($localfilename, 'wb');
$ch = curl_init();
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_FILE, $out);
curl_setopt($ch, CURLOPT_HEADER, 0);
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_USERPWD, "{$partnerid}:{$pass_hash}");
curl_exec($ch);
curl_close($ch);
fclose($out);
echo "Weno {$dir[$choice]}.zip downloaded!";
C# Code
using System;
using System.Net;
using System.Text;
namespace Weno
{
class Program
{
static void Main(string[] args)
{
// console application to download Weno directories
WebClientwebClient = new WebClient();
// available directories
string[] dir = { "DrugDBDirectory", "PharmacyDirectory", "PrescriberDirectory" };
// provide your Partner ID and password MD5 hash
// as shown on your dashboard under Account details -> Company Data
string partnerId = "123"; // partnerID here
string password = "XXXXXXXX"; // partner password md5 hash here
int choice = 0; // 0. Drugs, 1. Pharmacies, 2. Prescribers
// for live data use https://live.wenoexchange.com/wenox/GetListResponse.aspx instead
string url = "https://cert.wenoexchange.com/wenox/GetListResponse.aspx?" + dir[choice] + "=yes";
// authenticate with weno
webClient.Headers["Authorization"] = "Basic " + Convert.ToBase64String(Encoding.UTF8.GetBytes(partnerId + ":" + password));
webClient.Headers["Content-Type"] = "application/x-www-form-urlencoded";
// download file from weno
webClient.DownloadFile(new Uri(url), dir[choice] + ".zip");
}
}
}
Navigate to Manage Account/Clinic and add a test clinic. Then navigate to Manage Prescribers and add a test prescriber.
Use the test prescriber’s ID in the Header of your ePrescribing messages. The WENO assigned prescriber ID consists of a D and C value like this: D1234:C14332 in the From field of the Header.
Be sure to use the “D” qualifier.
D represents the prescriber and C represents his/her location. It is possible for a prescriber to have multiple locations.
If your EHR needs EPCS and you have your own DEA audit, provide this audit to admin@wenoexchange.com or your certification manager so your account will be enabled to route EPCS.
If your EHR will need EPCS and you do not have your own DEA audit yet, you should not use this API but the WENO Online one, as all EPCS NewRx will be rejected.
In a future step we will go over Manage APIs to add/edit/delete prescribers and locations. If you do not have many then we will allow the manual process as shown in this step.
Sample Real Time STATUS Response
IF HEADER OF THE MESSAGE RECEIVED FROM WENO SHOWS | THEN FROM IN THE HEADER OF YOUR REAL TIME STATUS HEADER WILL BE |
---|---|
<To>Jane Doe</To> | <From>Jane Doe</From> |
<To Qualifier=”D”>U12342:L97987</To> | <From Qualifier=”D”>U12342:L97987</From> |
<From>WENO</From> | <To>WENO</To> |
<From Qualifier=”P”>7654321</From> | <To Qualifier=”P”>7654321</To> |
Sample XML real-time status
<Message DatatypesVersion="20170715" TransportVersion="20170715" TransactionDomain="SCRIPT" TransactionVersion="20170715" StructuresVersion="20170715" ECLVersion="20170715"> <Header> <To Qualifier="D">UseOriginalSenderOfMessageHere</To> <From Qualifier="P">UseOriginalRecipientOfMessageHere</From> <MessageID>MustBEUnique</MessageID> <RelatesToMessageID>WillEchoOriginalMsgIDWithError</RelatesToMessageID> <SentTime>2019-06-29T09:49:07.70635Z</SentTime> <Security> <UsernameToken> <Username>UsernameToAuthenticateSendingSystem</Username> <Password Type="PasswordDigest">CredentialToAuthenticateSendingSystem</Password> </UsernameToken> </Security> <SenderSoftware> <SenderSoftwareDeveloper>AnyName</SenderSoftwareDeveloper> <SenderSoftwareProduct>SendingSystemName</SenderSoftwareProduct> <SenderSoftwareVersionRelease>V1</SenderSoftwareVersionRelease> </SenderSoftware> </Header> <Body> <Status> <Code>001</Code> <Description>Transaction successful</Description> </Status> </Body> </Message>
Sample JSON real-time status
{ "Message": { "@DatatypesVersion": "20170715", "@TransportVersion": "20170715", "@TransactionDomain": "SCRIPT", "@TransactionVersion": "20170715", "@StructuresVersion": "20170715", "@ECLVersion": "20170715", "Header": { "To": { "@Qualifier": "D", "#text": "UseOriginalSenderOfMessageHere" }, "From": { "@Qualifier": "P", "#text": "UseOriginalRecipientOfMessageHere" }, "MessageID": "MustBEUnique", "RelatesToMessageID": "WillEchoOriginalMsgIDWithError", "SentTime": "2019-06-29T09:49:07.70635Z", "Security": { "UsernameToken": { "Username": "UsernameToAuthenticateSendingSystem", "Password": "CredentialToAuthenticateSendingSystem" } }, "SenderSoftware": { "SenderSoftwareDeveloper": "AnyName", "SenderSoftwareProduct": "SendingSystemName", "SenderSoftwareVersionRelease": "V1" } }, "Body": { "Status": { "Code": "001", "Description": "Transaction successful" } } }
PHP Code to receive messages from WENO
// receive_from_weno.php - receive a message from weno service function listen() { $messageID = '0'; $now = date('Y-m-d H:i:s'); $body = trim(file_get_contents('php://input')); $MessageType = ($body[0] == '<') ? 'XML' : 'JSON'; LogMessage("<<< Incoming {$MessageType} at {$now}"); LogMessage($body); // echo incoming message from Switch if ($MessageType == 'XML') { $xmlBody=simplexml_load_string($body); LogMessage('PHP representation of message:'); LogMessage(print_r($xmlBody,true)); $messageID = $xmlBody->Header[0]->MessageID; $sFrom = $xmlBody->Header[0]->To; $sTo = $xmlBody->Header[0]->From; $response = GetStatusXML('000','Transaction Successful', '0', $sToQual, $sFromQual, $sTo, $sFrom); // send response to Switch print_r($response->asXML()); } else { $jsonBody = json_decode($body); //LogMessage('PHP representation of message:'); //LogMessage(print_r($jsonBody)); $sFrom = $jsonBody->Header->To; $sTo = $jsonBody->Header->From; $response = GetStatusJSON('000','Transaction OK','0', '','', $sTo, $sFrom); echo json_encode($response); } } function logMessage($line) { $filename = 'receive_log'; // log file $fp = fopen($filename, "a"); fwrite($fp, $line . PHP_EOL); fclose($fp); } function GetStatusXML($code, $msg, $messageID, $sToQual, $sFromQual, $sTo, $sFrom) { // // be sure you have realtime_status.xml on the specified path // $xml_path = './'; $response = simplexml_load_file("{$xml_path}realtime_status.xml"); $response->Body[0]->Status[0]->Code[0] = $code; $response->Body[0]->Status[0]->Description[0] = $msg; $response->Header[0]->From[0] = $sFrom; $response->Header[0]->From->attributes()->Qualifier = $sFromQual; $response->Header[0]->To[0] = $sTo; $response->Header[0]->To[0]->attributes()->Qualifier = $sToQual; $response->Header[0]->MessageID[0] = $messageID; $response->Header[0]->SentTime[0] = date('Y-m-d H:i:s'); //some time $response->Header[0]->RelatesToMessageID[0] = $messageID; return $response; } function GetStatusJSON($code, $msg, $messageID, $sToQual, $sFromQual, $sTo, $sFrom) { $json_path = './'; $response = json_decode(file_get_contents("{$json_path}realtime_status.json")); $response->Message->Body->Status->Code = $code; $response->Message->Body->Status->Description = $msg; $response->Message->Header->From->{'#text'} = $sFrom; $response->Message->Header->To->{'#text'} = $sTo; $response->Message->Header->MessageID = $messageID; $response->Message->Header->SentTime = date('Y-m-d H:i:s'); //some time $response->Message->Header->RelatesToMessageID = $messageID; return $response; } // listen for incoming messages listen();
C# Code to receive messages from WENO
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Xml; using System.IO; using System.Text; using System.Configuration; namespace SampleEndPoint { public class SampleEndpointBuiltFromSampleCode : IHttpHandler { public void ProcessRequest(HttpContext context) { context.Response.ContentType = "text/plain"; string response = WenoMessage(); context.Response.Write(response); } public string WenoMessage() { string response = ""; try { // To receive authorization info which is userid:password format string authStr = HttpContext.Current.Request.Headers["Authorization"].Replace("Basic ", ""); authStr = Encoding.UTF8.GetString(Convert.FromBase64String(authStr)); string messageID = "0"; MemoryStreamms = new MemoryStream(); // get message HttpContext.Current.Request.InputStream.CopyTo(ms); string xmlMessage = System.Text.Encoding.UTF8.GetString(ms.ToArray()); XmlDocumentxReq = new XmlDocument(); xReq.LoadXml(xmlMessage); // fetch message id string sMessagePath = @"/*[local-name() = 'Message']/*[local-name() = 'Header']/*[local-name() = 'MessageID']"; XmlNodemessNode = xReq.SelectSingleNode(sMessagePath); if (messNode != null) { messageID = messNode.InnerText; } string sFromPath = @"/*[local-name() = 'Message']/*[local-name() = 'Header']/*[local-name() = 'From']"; string sToPath = @"/*[local-name() = 'Message']/*[local-name() = 'Header']/*[local-name() = 'To']"; string sFromQual = ""; string sFrom = ""; string sToQual = ""; string sTo = ""; XmlNodefromNode = xReq.SelectSingleNode(sFromPath); XmlNodetoNode = xReq.SelectSingleNode(sToPath); if (fromNode != null &&toNode != null) { sFrom = fromNode.InnerText; sTo = toNode.InnerText; if (fromNode.InnerText.ToUpper().Contains("D")) { sFromQual = "D"; sToQual = "P"; } else { sFromQual = "P"; sToQual = "D"; } } string messType = "Unknown_Type"; string sMessageTypePath = @"/*[local-name() = 'Message']/*[local-name() = 'Body']"; if(xReq.SelectSingleNode(sMessageTypePath) != null) messType = xReq.SelectSingleNode(sMessageTypePath).ChildNodes[0].Name; // store/process message as per your requirement WriteFile(xmlMessage, messType + "_ " + DateTime.Now.ToString("yyyyMMddHHmmssfff") + ".xml"); string[] arrAuth = authStr.Split(new char[] { ':' }); if (arrAuth.Length == 2) { string reqUserID = arrAuth[0]; string reqPassword = arrAuth[1]; string ClientUserID = ConfigurationManager.AppSettings["UserID"]; string ClientPassword = ConfigurationManager.AppSettings["Password"]; // send success/failure message in xml format as specified // To note here that in response to becomes from and from becomes to // If you know you are a pharmacy or ehr you can set hardcoded values here //response = GetStatusXmlFromWeno2017071("001", "Transaction Successfull", "", "M", "WENO"); //you can authenticate request as well if (reqUserID == ClientUserID&&reqPassword == ClientPassword) { response = GetStatusXmlFromWeno2017071("001", "Transaction Successfull", "", "M", "WENO"); } else { response = GetErrorXmlFromWeno2017071("900","Authentication Failed","","M","WENO", Guid.NewGuid().ToString().Replace("-", "")); } } else { response = GetErrorXmlFromWeno2017071("900", "Invalid request", "", "M", "WENO", Guid.NewGuid().ToString().Replace("-", "")); } } catch (System.Exception ex) { response = GetErrorXmlFromWeno2017071("900", "Unhandled Error", "", "M", "WENO", Guid.NewGuid().ToString().Replace("-", "")); } //string sFinalResponse = Convert.ToBase64String(Encoding.UTF8.GetBytes(response)); return response; } public static void WriteFile(string strContent, string fileName) { FileStream fs = null; FileInfo fi = new FileInfo(AppDomain.CurrentDomain.BaseDirectory + "\\Messages\\" + fileName); if (!fi.Directory.Exists) { fi.Directory.Create(); } fs = new FileStream(fi.FullName, FileMode.Create); StreamWritersw = new StreamWriter(fs); sw.WriteLine(strContent); sw.Close(); fs.Close(); } public static string GetStatusXml1(string errorCode, string description, string messageID, bool IsError,stringfromQual = "",string toQual = "", string from = "", string to = "") { XmlDocumentxmlTemplate = new XmlDocument(); if (!IsError) { xmlTemplate.Load(AppDomain.CurrentDomain.BaseDirectory + "\\xmlTemplate\\Status.xml"); } else { xmlTemplate.Load(AppDomain.CurrentDomain.BaseDirectory + "\\xmlTemplate\\Error.xml"); } XmlNodeheaderXmlTemplate = xmlTemplate.GetElementsByTagName("Header")[0]; headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.FromQualifier}", fromQual); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.From}", from); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.ToQualifier}", toQual); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.To}", to); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.MessageID}", "0"); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.SentTime}", DateTime.Now.ToUniversalTime().ToString("yyyy-MM-ddTHH:mm:ss.fffffK")); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.RelatesToMessageID}", messageID); XmlNodebodyXmlTemplate = xmlTemplate.GetElementsByTagName("Body")[0]; bodyXmlTemplate.InnerXml = bodyXmlTemplate.InnerXml.Replace("{Status.Code}", errorCode); bodyXmlTemplate.InnerXml = bodyXmlTemplate.InnerXml.Replace("{Status.Description}", description); return xmlTemplate.InnerXml; } public static string GetStatusXmlFromWeno2017071(string errorCode, string description, string descriptionCode, string toQualifier, string to) { XmlDocumentxmlTemplate = new XmlDocument(); xmlTemplate.Load(AppDomain.CurrentDomain.BaseDirectory + "\\xmlTemplate\\Status2017071.xml"); XmlNodeheaderXmlTemplate = xmlTemplate.GetElementsByTagName("Header")[0]; headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.FromQualifier}", "M"); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.From}", "WENO"); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.ToQualifier}", toQualifier); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.To}", to); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.MessageID}", "0"); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.SentTime}", DateTime.Now.ToUniversalTime().ToString("yyyy-MM-ddTHH:mm:ss.fffffK")); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Status.RelatesToMessageID}", "0"); XmlNodebodyXmlTemplate = xmlTemplate.GetElementsByTagName("Body")[0]; bodyXmlTemplate.InnerXml = bodyXmlTemplate.InnerXml.Replace("{Status.Code}", errorCode); bodyXmlTemplate.InnerXml = bodyXmlTemplate.InnerXml.Replace("{Status.DescriptionCode}", descriptionCode); bodyXmlTemplate.InnerXml = bodyXmlTemplate.InnerXml.Replace("{Status.Description}", description); return xmlTemplate.InnerXml; } public static string GetErrorXmlFromWeno2017071(string errorCode, string description, string descriptionCode, string toQualifier, string to, string MessageID = "0", string RelatesToMessageID = "0") { XmlDocumentxmlTemplate = new XmlDocument(); xmlTemplate.Load(AppDomain.CurrentDomain.BaseDirectory + "\\xmlTemplate\\Error2017071.xml"); XmlNodeheaderXmlTemplate = xmlTemplate.GetElementsByTagName("Header")[0]; headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Error.FromQualifier}", "M"); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Error.From}", "WENO"); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Error.ToQualifier}", toQualifier); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Error.To}", to); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Error.MessageID}", MessageID); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Error.SentTime}", DateTime.Now.ToUniversalTime().ToString("yyyy-MM-ddTHH:mm:ss.fffffK")); headerXmlTemplate.InnerXml = headerXmlTemplate.InnerXml.Replace("{Error.RelatesToMessageID}", RelatesToMessageID); XmlNodebodyXmlTemplate = xmlTemplate.GetElementsByTagName("Body")[0]; bodyXmlTemplate.InnerXml = bodyXmlTemplate.InnerXml.Replace("{Error.Code}", errorCode); bodyXmlTemplate.InnerXml = bodyXmlTemplate.InnerXml.Replace("{Error.DescriptionCode}", descriptionCode); bodyXmlTemplate.InnerXml = bodyXmlTemplate.InnerXml.Replace("{Error.Description}", description); return xmlTemplate.InnerXml; } public bool IsReusable { get { return false; } } } }
TEST YOUR ENDPOINT
This step will be marked completed when you successfully return STATUS message back to WENO using the endpoint testing tool found here MANAGE ACCOUNT -> MANAGE IPS AND ENDPOINTS. Note: when asked for the endpoint URL be sure to specify the full path of the endpoint URL containing the script that handles the messages Weno sends to your endpoint. For example, if your server is located at https://www.myehr.com and the endpoint script is under /endpoint.php then your endpoint URL is https://www.myehr.com/endpoint.php Weno also provides an Endpoint Validation Tool to verify your endpoint. On step 1, when asked to enter a secure endpoint just insert your secure (https) endpoint URL which includes the endpoint handler/script location. Using the previous example this would be https://www.myehr.com/endpoint.php You can test your endpoint by sending yourself our samples or send your own sample messages. This image shows how this is done in your Manage IP and Endpoint page.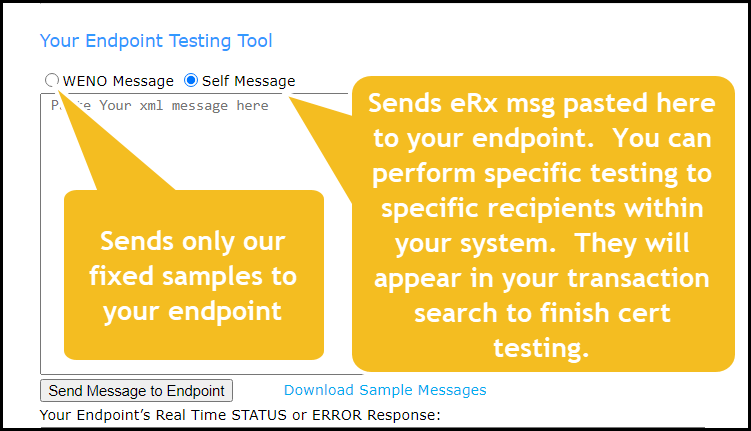
This step is to teach you the communication flow which happens when you send valid ePrescribing messages. Error handling and Manage APIs messages are covered in another step. How to send these are in Step 6.
Access the Schemas you need here
NCPDP 20170715: This is the official government standard NCPDP schema for ePrescribing. It is a huge schema that has 49 type of ePrescribing and specialized eRx messages and requires the use of referencing schemas. For your sanity we have provided a mini schema of the NewRx.
NewRx Mini Schema: simplified schema with most common message types to get you started.
WENO NONCE Schema: this is the schema you will use to send WENO Switch ePrescribing messages.
NewRx Validation and recommended use
Some validations are based on the schema’s standards and others are not real validations but come from a NCPDP standard recommendation or a state rule. The pharmacies can reject messages that do not conform to their state rule or a NCPDP standard recommendation.
Rule | Comment |
NCPDP 20170715 schema | WENO has a validator tool on the login page of your cert dashboard. |
Partner Authentication | Nonce use |
Whitelist IP | Weno verifies IPs are registered |
SentTime UTC | Standards require UTC |
WrittenDateTime in UTC | Populate WrittenDateTime(not just WrittenDate) in UTC or pharmacies may reject. |
Prescriber Suffix | Suffix is the prescriber’s credentials. Like MD, DO, etc. Many states require. |
Supervisor Suffix | If prescriber is a midlevel, when you populate Supervisor make sure you populate the suffix. |
Midlevel requires Supervisor Segment | A midlevel prescriber, like an RN needs a supervising prescriber listed. |
Days Supply | Required in many states so always populate or eRxs can be rejected |
DEA schedule | You must populate this with the proper DEA NCIT code found in Weno drug database, your commercial drug database or by using RxNorm. |
Potency Unit Code | This code is extremely important. Found in WENO’s drug database file. It is the only way to understand the dosing units for the quantity field. Tablets, milliliters, eaches, packets, etc. The prescriber will not understand how a drug is packaged and measured per unit unless you show it to them. |
Effective Date | You should use the Effective Date as shown in the NewRx sample when the prescriber does not want the drug dispensed right away (in the future). |
Notes | This is for a prescriber to post a note specifically to the pharmacist and the pharmacist can reject eRxs that use this field for other purposes. |
Patient’s 18 years or under | Must have height and weight as shown in NewRx sample |
Digital Signature Indicator in Header or NewRx | If you have an EPCS audit and the prescriber digitally signed the prescription mark TRUE otherwise FALSE |
Message ID in NewRx Header | Must be unique |
D qualifier in TO/FROM field of header | If you populate the TO or FROM “qualifier” field with a D then we expect the prescriber full WENO Prescriber ID (combination of D and C value which represents Prescriber and Location ID as registered). To find use Manage APIs or go to Manage Account. |
C qualifier in TO/FROM Field of NewRx Header | If you populate the qualifier with a C then WENO is expecting the prescriber’s Location value in the VALUE. Example: C23423 |
Pharmacy Fax | If you have it send it as our failover process is faxing and we have a fax # but if yours is different we will automatically use ours then try yours. |
Diagnosis | Some states require this field. The NewRx sample shows how to populate it. |
Prescriber Place Of Service | We do not validate, but many states require the place of service populated so we recommend it to avoid rejections. Allowed values for this field are here |
The Header of all NCPDP Standard 20170715 Messages Explained
Header Field | Requirement & Cardinality Notes | Data Type | Value Comments |
Message Attributes | Yes, occurs 1x. | an | DatatypesVersion=”20170715″ TransportVersion=”20170715″ TransactionDomain=”SCRIPT” TransactionVersion=”20170715″ StructuresVersion=”20170715″ ECLVersion=”20170715″ |
TO Qualifier | Yes, occurs 1x. Required by WENO, not by the standard schema, so message may validate against the schema but WENO will reject because they cannot identify the TO value. | ECL codes | The ECL codes options WENO support are as follows: P = Pharmacy D = Prescriber (if you populate the qualifier with “D” then WENO is expecting D value and C value combined to make the prescriber’s ID. For example: D13242:C098) C = Clinic (then the value WENO expects is L value for the prescriber’s location as registered) CF = Central Fill Pharmacy ZZZ = Mutually Defined PY = Payer REMS = REMS Administrator |
TO | Yes, occurs 1x. | an 1..255 | The WENO Directory will provide the necessary IDs below: If P is TO Qualifier then enter NCPDP ID If D is TO Qualifier enter WENO prescriber ID (see comments above) If C is TO Qualifier enter WENO Clinic ID (see comments above) If CF is TO Qualifier enter the WENO Central Fill Pharmacy ID If ZZZ is TO Qualifier enter the appropriate WENO ID If PY is TO Qualifier enter the WENO Payer ID IF REMS is TO Qualifier enter the WENO REMS Administrator ID |
From Qualifier | Yes, occurs 1x. | ECL codes | Expected values: P = Pharmacy D = Prescriber WENO ID C = Clinic value for prescriber location as registered CF = Central Fill Pharmacy ZZZ = Mutually Defined PY = Payer REMS = REMS Administrator |
From | Yes, occurs 1x. | an 1..255 | If the From Qualifier is blank – enter the prescriber name or NPI; otherwise populate as follows: If P is TO Qualifier then enter NCPDP ID If D is TO Qualifier enter WENO prescriber ID (see comments above) If C is TO Qualifier enter WENO Location ID as registered If CF is TO Qualifier enter the WENO Central Fill Pharmacy ID If ZZZ is TO Qualifier enter the appropriate WENO ID If PY is TO Qualifier enter the WENO Payer ID IF REMS is TO Qualifier enter the WENO REMS Administrator ID The WENO Directory will provide the necessary IDs. |
Message ID | Yes, occurs 1x. | an 1..35 | Trace number. A unique reference identifier for the transmission, generated from the sender of the request and the sender of the response. When generated from the sender, it is then echoed back in the response message in the field RelatesToMessageID. The value in this field must be present in RelatesToMessageID on subsequent transactions (such as RxRenewalRequest, CancelRx, etc) to tie back to an original transmission. IF STATUS or ERROR msg are a real-time response, the the message ID can either be zero or a unique ID. |
RelatesToMessageID | NO, occurs 1x if used. This is needed to tie back to a previous transaction | An 1..35 | On a direct response transaction (such as Error, Status, RxHistoryResponse) or a subsequent follow up response (such as RxChangeResponse, RxRenewalResponse, CancelRxResponse, MTMServiceResponse) or a subsequent follow up transaction (such as RxFill, Verify), this field is mandatory and is used to link the original message (MessageID) from request to the response or to the subsequent follow up transaction. |
Sent Time | Yes, occurs 1x. | UTC Date Type | UTCTimeCode (example: 1970-11-03T13:10:30.64) |
Security | Yes, occurs 1x. Required by WENO to authenticate API partner | Security, UsernameToken, Username = required, an1…35, occurs 1x; Security, UsernameToken, Password Attribute= Required, Fixed “PasswordDigest”, occurs 1x. Security, UsernameToken, Password= Required, string, occurs 1x. Security, UsernameToken, Nonce = not required, occurs 1x if used. Security, UsernameToken, Created= not required, UTC Date Type, occurs 1x if used. SecondaryIdentification= for prescriber username, an1…35, occurs 1x if used. TertiaryIdentification = for prescriber MD5 hash of password, an1…35, occurs 1x if used | WENO will soon require NONCE value to replace API Partner ID and password. Currently Minimal requirements for the Security of the Header is as follows:<Security> <UsernameToken> <Nonce>1032894770982709870</Nonce> </UsernameToken> <Security> |
SenderSoftware, SenderSoftwareDeveloper | Yes, occurs 1x | an 1..35 | A contact person for troubleshooting |
SenderSoftware, SenderSoftwareProduct | Yes, occurs 1x | an 1..35 | Identifies the entity responsible that generated the transaction. Note: For Status, Error, Verify transactions – contains software identification of the entity creating the response. |
SenderSoftware, SenderSoftwareVersionRelease | Yes, occurs 1x | an 1..50 | Identifies the software product version release of the entity responsible for generating the transaction or creating the response. |
Mailbox | NO | NOT supported- do not populate | GET Message and Mailboxing is not supported by WENO |
TestMessage | NO, occurs 1x if used | Boolean | Indicates if the message is a test message; however WENO does not allow test messages to go to real entities without prior approval from WENO. WENO provides test Pharmacies, Prescriber and other types of accounts for testing in the WENO Directory. |
RxReferenceNumber | NO, occurs 1x if used | an 1..35 | Not used by WENO – you may populate if it is useful |
TertiaryIdentifier | NO, occurs 1x if used | an 1..3 | Not used by WENO – you may populate if it is useful |
PrescriberOrderNumber | NO, occurs 1x if used | an 1..35 | Not used by WENO – you may populate if it is useful |
DigitalSignature Version attribute | Yes, occurs 1x. | an | In order to send EPCS orders an EHR must have its DEA Audit sent to admin@wenoexchange.com. After that, populate the DigitalSignature Version attribute with the version your EHR users use to digitally sign the EPCS orders. If your EHR does not have its own DEA Audit you set the Version attribute to “not applicable” |
DigitalSignatureIndicator | Yes, occurs 1x. | Boolean | If your EHR has its own DEA Audit set as 1 else as 0 |
PrescriberOrderGroup | NO, occurs 1x if used. | See image in the next column for data types if used. | Not used by WENO – you may populate if it is useful |
RxOrderReferenceGroup | NO, occurs 1x if used | See image in the next column for data types if used. | Not used by WENO – you may populate if it is useful |
Using NONCE
A NONCE is a key specific to a message ID. Using the NONCE in a message will avoid the need of using partner and user credentials and therefore it improves the overall security. Prescribers need to generate a NONCE each time they send a new message.
Steps to use NONCE instead of Partner/User credentials on WENO Switch
- Partner will first need to call WenoSwitchNonceID at the endpoint with a SwitchNonceRequest XML that complies with the NonceSchema schema.
SwitchNonceRequest request contains authentication info (Partner ID/password) along with Message ID. If the credentials are successfully verified then a SwitchNonceResponse containing the NONCE is returned. - Weno_Switch NONCE returned on step 1 should be plugged in the eRx XML message. Credentials are not needed.
Sample header of NewRx using Nonce
<Header> <To Qualifier="P">7654321</To> <From Qualifier=”D”>D13242:C098</From> <MessageID>MustBeUnique</MessageID> <SentTime>2020-02-11T04:56:15.45</SentTime> <Security> <UsernameToken> <Nonce>EnterYourNonceKey</Nonce> </UsernameToken> </Security> <SenderSoftware> <SenderSoftwareDeveloper>EnterName</SenderSoftwareDeveloper> <SenderSoftwareProduct>Enter Your Software's Name</SenderSoftwareProduct> <SenderSoftwareVersionRelease>V1</SenderSoftwareVersionRelease> </SenderSoftware> <DigitalSignature Version="F"> <DigitalSignatureIndicator>1</DigitalSignatureIndicator> </DigitalSignature> </Header>
The Body of the NewRx Message Explained
Body/NewRx Field | DataType | Max Uses | Comments |
MessageRequestCode | string pattern | 0..1 | For Long Term Care Use – Enter the LTC level of change code here “C1” Label change (Any changes to the Drug, form, strength, dosage, or route) – Change to an active order to the drug, form, strength, dosage, or route (long term care settings). “C2” Frequency Change (Any change to the frequency or hours of administration for the drug) – Change to the frequency or hours of administration for the medication (long term care settings). “C3″Other Change (All other changes) – A change to the medication not covered by other values listed (long term care settings). “OS” Pharmacy is out of stock of the medication prescribed and it cannot be obtained in a clinically appropriate timeframe |
ReturnReceipt | an 1..3 | 0..1 | Enter “1” if your system wants WENO to return the message “VERIFY” when the transmission was successfully sent to the recipient. |
RequestReferenceNumber | an1..35 | 0..1 | Do not use – it only used for MAILBOXING (WENO does not support this legacy communication method for ePrescribing) |
UrgencyIndicatorCode | string pattern | 0..1 | X – Urgent/Expedited S – Standard |
FollowUpRequest | – | 0..0 | Ignore – not used in NewRx. It will cause validation issues with NewRx. |
ChangeOfPrescriptionStatusFlag | – | 0..0 | Ignore – not used in NewRx. It will cause validation issues with NewRx. |
AllergyOrAdverseEvent | Type | 0..1 | This is a complex type that allows you to indicate either NoKnownAllergies or any number of loops of allergy information. Refer to the schema if you are planning to populate this information in the NewRx. |
BenefitsCoordination | Type | 0..4 | This is a complex type. Refer to schema or WENO’s examples if you will populate this field to receive free ePrescribing. |
DiagnosisGeneral | – | 0..0 | Do not use – it will not validate. It is a field for long term care….see schema for more information. The MedicationPrescribed Segment has a place for prescribers to enter the patient Diagnosis. |
Facility | Type | 0..1 | Refer to schema |
FillStatus | – | 0..0 | Do not use – it will not validate |
Patient | Type | 1 | Choice between Human and NonHuman Patient. This is a complex type. Refer to schema to understand how to populate all fields. These are the most logical fields to populate for Human Patient:<Patient> <HumanPatient> <Name> <LastName>up to 35 an characters</LastName> <FirstName>up to 35 an characters</FirstName> </Name> <Gender>Use M, F, or U</Gender> <DateOfBirth> <Date>1955-06-17</Date> </DateOfBirth> <Address> <AddressLine1>Add up to 40 characters </AddressLine1> <AddressLine2> up to 40 characters to use if address line 1 is not enough </AddressLine2> <City>Austin</City> <StateProvince>TX</StateProvince> <PostalCode>78726</PostalCode> <CountryCode>US</CountryCode> </Address> <CommunicationNumbers> <PrimaryTelephone> <Number>if you have it send it</Number> <SupportsSMS>T or F for True/False</SupportsSMS> </PrimaryTelephone> </CommunicationNumbers> </HumanPatient> </Patient>These are the most logical fields to populate for NON-Human Patient: <NonHumanPatient> <Name> <LastName>Use the last name of owner here</LastName> <FirstName>This is the animal name</FirstName> </Name> <Gender>M</Gender> <DateOfBirth> <Date>2015-01-12 – use best guess for this</Date> </DateOfBirth> <Species> <Text>Coonhound (this must be exactly as code describes below)</Text> <Code>73319009 (this is a SnowMed code)</Code> </Species> </NonHumanPatient> |
Pharmacy | Type | 0..1 | <Pharmacy> <Identification> <NCPDPID>an..35 characters to add pharmacy’s NCPDP ID</NCPDPID> <NPI>an..35 characters to populate NPI if you have it on the WENO directory - download and update your WENO directory daily or weekly to find NCPDP IDs and NPIs </NPI> <MutuallyDefined>use this for international pharmacy IDs if applicable</MutuallyDefined> </Identification> <BusinessName>an..35 characters for pharmacy store name</BusinessName> <Address> <AddressLine1>up to 40 characters -if you have it send it</AddressLine1> <AddressLine2>up to 40 characters if line 1 is not enough</AddressLine2> <City>string</City> <StateProvince>TX</StateProvince> <PostalCode>78788</PostalCode> <CountryCode>US</CountryCode> </Address> <CommunicationNumbers> <PrimaryTelephone> <Number>n..10, if you have it send it</Number> </PrimaryTelephone> </CommunicationNumbers> <CommunicationNumbers> <Fax> <Number>provide if you have it n..10</Number> </Fax> </CommunicationNumbers> </Pharmacy> |
Prescriber | Type | 1 | You have the choice of Veterinarian and NonVeterinarian prescriber segments. For <PrescriberPlaceOfService> accepted values please refer to this link. For all the possible fields to populate refer to the schema. This example shows the most commonly populated fields for each type. Non Veterinarian <Prescriber> <NonVeterinarian> <Identification> <StateLicenseNumber>0987098707</StateLicenseNumber> <DEANumber>123</DEANumber> <NPI>1234567890</NPI> </Identification> <Name> <LastName>Doright</LastName> <FirstName>Dudley</FirstName> <Suffix>NP</Suffix> </Name> <Address> <AddressLine1>123 Main Street</AddressLine1> <City>Austin</City> <StateProvince>TX</StateProvince> <PostalCode>78726</PostalCode> <CountryCode>US</CountryCode> </Address> <CommunicationNumbers> <PrimaryTelephone> <Number>2228889999</Number> </PrimaryTelephone> </CommunicationNumbers> <PrescriberPlaceOfService>49<PrescriberPlaceOfService> </NonVeterinarian> </Prescriber>For Veterinarian <Prescriber> <Veterinarian> <Identification> <StateLicenseNumber>TestLicenseNo1233</StateLicenseNumber> </Identification> <Specialty>Use 73M00000X for Veterinarian or 174MM1900X for Medical Research Vet.</Specialty> <Name> <LastName>Smith</LastName> <FirstName>Tom</FirstName> <MiddleName>E</MiddleName> <Suffix>VMD</Suffix> </Name> <Address> <AddressLine1>1231 Tall Grass Court</AddressLine1> <City>Napa</City> <StateProvince>CA</StateProvince> <PostalCode>94558</PostalCode> </Address> <CommunicationNumbers> <PrimaryTelephone> <Number>7190072303</Number> </PrimaryTelephone> </CommunicationNumbers> <PrescriberPlaceOfService>49<PrescriberPlaceOfService> </Veterinarian> </Prescriber> |
DispensingRequestCode | – | 0..0 | Do not use – it will not validate |
DrugAdministrationRequest | – | 0..0 | Do not use – it will not validate |
Observation | Type | Cond 0..1 | Height and Weight are required when patient is human 18 years of age or under. It can be added as a text field in the ObservationNotes instead of the Measurement field which allows for 10 loops. <Observation> <Measurement> <VitalSign>Weight</VitalSign> <LOINCVersion>2.64</LOINCVersion> <Value>112</Value> <UnitOfMeasure>pounds</UnitOfMeasure> <UCUMVersion>2.1</UCUMVersion> <ObservationDate> <Date>2020-01-05</Date> </ObservationDate> </Measurement> <Measurement> <VitalSign>Height</VitalSign> <LOINCVersion>2.66</LOINCVersion> <Value>62</Value> <UnitOfMeasure>inches</UnitOfMeasure> <UCUMVersion>2.1</UCUMVersion> <ObservationDate> <Date>2020-01-05</Date> </ObservationDate> </Measurement> <ObservationNotes>patient is 62 inches in height and weighs 112 lbs </ObservationNotes> </Observation> |
MedicationDispensed | – | 0..0 | DO Not Use – it will not validate |
MedicationPrescribed | Type | 1 | This is an extremely complex type. WENO has included information on commonly populated fields, but there are many to use because prescribers can prescribe very complex or simple medication. This segment has a place to enter the diagnosis and a place for “Other Medication Date” which can record an Effective date when the prescriber does not intend the prescription to be filled right away. The diagnosis and Other Medication Date fields are not in this example. Use the schema to discover the fields that can be populated or contact WENO if you need more help with this segment of the NewRx.<MedicationPrescribed> <DrugDescription> Required field. an…105 enter drug name strength and form from PSN column of WENO Drugdatabase, or in case of a compound the name or nickname of the compound and then populate the Compound segment which is not shown in this example. Refer to schema </DrugDescription> <DrugCoded> <ProductCode> <Code>Not required but if used, this is the place for another type of drug code when an RX Norm CUI code or a drug code from a commercial data base is not available, typically this is an NDC code.</Code> <ProductQualifierCode> Not required but if Product Code/Code is populated then it must be populated to qualify where the non-drug database code came from, see ECL of the schema for the qualifier code values. If the code is an NDC then the value here is “ND”. </ProductQualifierCode> </ProductCode> <DrugDBCode> <Code> Not required but recommended. If you are using WENO's Drug database the RX Norm CUI code goes here if there is one, while DrugDBCode is not required, it should certainly be used as the drug description is in text form and not database friendly, so the prescriber may get pharmacy calls. If you use a commercial drug database their code goes here. </Code> <Qualifier> Must ID the drug database the drug code came from - from the list of acceptable ones see ECL. If you are using WENO drug database then this is the TTY value. </Qualifier> </DrugDBCode> <DEASchedule> <Code> Required. WENO will reject the message if you do not populate this with the appropriate code. We include them here but they are also mapped on the WENO drug database: C48672 is code for DEA Schedule I drugs but these are illegal to prescribe C48675 is code for DEA schedule II drugs C48676 is code for DEA schedule III drugs, C48677 is code for DEA schedule IV drugs C48679 is code for DEA schedule V drugs, and C38046 is code for DEA unspecified or when a drug has no specified DEA schedule. </Code> </DEASchedule> </DrugCoded> <Quantity> <Value>Required n1..11</Value> <CodeListQualifier>Required, enter “38” as the code here always, it means original quantity</CodeListQualifier> <QuantityUnitOfMeasure> <Code> Required, enter the potency unit code from WENO's drug database here</Code> </QuantityUnitOfMeasure> </Quantity> <DaysSupply>30</DaysSupply> <WrittenDate> <DateTime>2020-04-23T12:00:05.16</</DateTime> </WrittenDate> <Substitutions>Enter value “1” if prescriber allows substitutions and "0" if not</Substitutions> <NumberOfRefills>n1..2</NumberOfRefills> <Note>Not required. 210 an characters - hardcode WENO's RX Savings Card info here if you elect free ePrescribing</Note> <Sig> <SigText> Required field. Enter up to 1000 an characters (not machine readable ones) for the drug's administration directions - this is not for pharmacy notes. For complex compounds and administration instructions the Sig section expands in the schema - see schema. </SigText> </Sig> <OtherMedicationDate> <OtherMedicationDate> <Date>2020-05-15</Date> </OtherMedicationDate> <OtherMedicationDateQualifier>EffectiveDate</OtherMedicationDateQualifier> </OtherMedicationDate> </MedicationPrescribed> |
MedicationRequested | – | 0..0 | Do not use – it will not validate. |
Supervisor | Type | 0..1 | See sample – it needs the same data as the prescriber populated, including suffix |
RequestedDates | – | 0..0 | Do not use – it will not validate |
ProhibitRenewalRequest | Boolean | 0..1 | Enter 0 if refill requests are not allowed by prescriber and Enter 1 if they are. |
ChangeReasonText | – | 0..0 | Do not use – it will not validate |
FollowUpPrescriber | Type | 0..1 | See schema if you plan to populate this. |
PostRxNonceRequest Sample
Please note the values provided are only fictitious. You will have to provide valid ones.<Message xmlns="https://wenoexchange.com/schema/RXNONCE"> <Header> <SentTime>2020-10-26T02:44:09</SentTime> </Header> <Body> <SwitchNonceRequest> <AuthenticationInfo> <APIPartnerID>1206</APIPartnerID> <APIPartnerPassword>86F7E437FAA5A7FCE15D1DDCB9EAEAEA377667B8</APIPartnerPassword> </AuthenticationInfo> <RxMessageId>a77d77d1850a492c9e5209387b367deb</RxMessageId> </SwitchNonceRequest> </Body> </Message>
A valid NewRx Sample
<?xml version="1.0" encoding="utf-8"?> <Message xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" DatatypesVersion="20170715" TransportVersion="20170715" TransactionDomain="SCRIPT" TransactionVersion="20170715" StructuresVersion="20170715" ECLVersion="20170715"> <Header> <To Qualifier="P">7654321</To> <From Qualifier="D">D101598:C29792</From> <MessageID>MustBeUnique</MessageID> <SentTime>2020-02-11T04:56:15.45</SentTime> <Security> <UsernameToken> <Nonce>9609879876987698769769</Nonce> </UsernameToken> </Security> <SenderSoftware> <SenderSoftwareDeveloper>SmartGuy</SenderSoftwareDeveloper> <SenderSoftwareProduct>Best EHR Ever</SenderSoftwareProduct> <SenderSoftwareVersionRelease>V1</SenderSoftwareVersionRelease> </SenderSoftware> <DigitalSignature Version="Not Applicable"> <DigitalSignatureIndicator>0</DigitalSignatureIndicator> </DigitalSignature> </Header> <Body> <NewRx> <ReturnReceipt>001</ReturnReceipt> <AllergyOrAdverseEvent> <NoKnownAllergies>Y</NoKnownAllergies> </AllergyOrAdverseEvent> <BenefitsCoordination> <PayerIdentification> <ProcessorIdentificationNumber>HT</ProcessorIdentificationNumber> <IINNumber>011867</IINNumber> </PayerIdentification> <CardholderID>Patient Phone or ZIP</CardholderID> <GroupID>BSURE+PartnerID</GroupID> <PayerType>L</PayerType> </BenefitsCoordination> <BenefitsCoordination> <PayerIdentification> <ProcessorIdentificationNumber>BBHT</ProcessorIdentificationNumber> <IINNumber>02342315284</IINNumber> </PayerIdentification> <CardholderID>3423423</CardholderID> <GroupID>MR455</GroupID> <PayerType>D</PayerType> </BenefitsCoordination> <Patient> <HumanPatient> <Name> <LastName>Doe</LastName> <FirstName>Jane</FirstName> </Name> <Gender>F</Gender> <DateOfBirth> <Date>2004-06-17</Date> </DateOfBirth> <Address> <AddressLine1>HN 321, Main Road</AddressLine1> <City>Austin</City> <StateProvince>TX</StateProvince> <PostalCode>78726</PostalCode> <CountryCode>US</CountryCode> </Address> <CommunicationNumbers> <PrimaryTelephone> <Number>2223334444</Number> <SupportsSMS>Y</SupportsSMS> </PrimaryTelephone> </CommunicationNumbers> </HumanPatient> </Patient> <Pharmacy> <Identification> <NCPDPID>7654321</NCPDPID> <NPI>34537654321</NPI> </Identification> <Specialty>Retail</Specialty> <BusinessName>Test Stand Alone Pharmacy</BusinessName> <Address> <AddressLine1>22 Main</AddressLine1> <AddressLine2>Madhya Marg</AddressLine2> <City>Austin</City> <StateProvince>TX</StateProvince> <PostalCode>78759</PostalCode> <CountryCode>US</CountryCode> </Address> <CommunicationNumbers> <PrimaryTelephone> <Number>8988989989</Number> </PrimaryTelephone> <Fax> <Number>8003662027</Number> </Fax> </CommunicationNumbers> </Pharmacy> <Prescriber> <NonVeterinarian> <Identification> <StateLicenseNumber>0987098707</StateLicenseNumber> <DEANumber>123</DEANumber> <NPI>1234567890</NPI> </Identification> <Name> <LastName>Doright</LastName> <FirstName>Dudley</FirstName> <Suffix>NP</Suffix> </Name> <Address> <AddressLine1>123 Main Street</AddressLine1> <City>Austin</City> <StateProvince>TX</StateProvince> <PostalCode>78726</PostalCode> <CountryCode>US</CountryCode> </Address> <CommunicationNumbers> <PrimaryTelephone> <Number>2228889999</Number> </PrimaryTelephone> </CommunicationNumbers> <PrescriberPlaceOfService>49</PrescriberPlaceOfService> </NonVeterinarian> </Prescriber> <Observation> <Measurement> <VitalSign>Weight</VitalSign> <LOINCVersion>2.64</LOINCVersion> <Value>112</Value> <UnitOfMeasure>pounds</UnitOfMeasure> <UCUMVersion>2.1</UCUMVersion> <ObservationDate> <Date>2020-01-05</Date> </ObservationDate> </Measurement> <Measurement> <VitalSign>Height</VitalSign> <LOINCVersion>2.66</LOINCVersion> <Value>62</Value> <UnitOfMeasure>inches</UnitOfMeasure> <UCUMVersion>2.1</UCUMVersion> <ObservationDate> <Date>2020-01-05</Date> </ObservationDate> </Measurement> <ObservationNotes>patient is 62 inches in height and weighs 112 lbs </ObservationNotes> </Observation> <MedicationPrescribed> <DrugDescription>Lipitor 10 MG Oral Tablet</DrugDescription> <DrugCoded> <DrugDBCode> <Code>854875</Code> <Qualifier>SBD</Qualifier> </DrugDBCode> <DEASchedule> <Code>C38046</Code> </DEASchedule> </DrugCoded> <Quantity> <Value>30</Value> <CodeListQualifier>38</CodeListQualifier> <QuantityUnitOfMeasure> <Code>C48542</Code> </QuantityUnitOfMeasure> </Quantity> <DaysSupply>30</DaysSupply> <WrittenDate> <DateTime>2020-02-11T01:56:15.45</DateTime> </WrittenDate> <Substitutions>0</Substitutions> <NumberOfRefills>0</NumberOfRefills> <Diagnosis> <ClinicalInformationQualifier>1</ClinicalInformationQualifier> <Primary> <Code>G47.00</Code> <Qualifier>ABF</Qualifier> <Description>Insomnia</Description> </Primary> </Diagnosis> <Note>patient likes a certain generic please accommodate if you can</Note> <Sig> <SigText>Take one tablet before bedtime</SigText> </Sig> <OtherMedicationDate> <OtherMedicationDate> <Date>2020-03-15</Date> </OtherMedicationDate> <OtherMedicationDateQualifier>EffectiveDate</OtherMedicationDateQualifier> </OtherMedicationDate> </MedicationPrescribed> <Supervisor> <NonVeterinarian> <Identification> <StateLicenseNumber>09987987</StateLicenseNumber> <DEANumber>12344</DEANumber> <NPI>234212890</NPI> </Identification> <Name> <LastName>Johnson</LastName> <FirstName>Tom</FirstName> <Suffix>MD</Suffix> </Name> <Address> <AddressLine1>123 Main Street</AddressLine1> <City>Austin</City> <StateProvince>TX</StateProvince> <PostalCode>78726</PostalCode> <CountryCode>US</CountryCode> </Address> <CommunicationNumbers> <PrimaryTelephone> <Number>2228889999</Number> </PrimaryTelephone> </CommunicationNumbers> </NonVeterinarian> </Supervisor> </NewRx> </Body> </Message>
- Endpoint: https://cert.wenoexchange.com/wenox/service.asmx
- Web method for posting Rx: wenoswitch
Test Pharmacies
We flag test pharmacies but these will give you desired results. Practice sending to these pharmacies using P as qualifier in TO field and these NCPDP IDs which will provide normal or certain errors. We cover errors in a future step.
Pharmacy Name | NCPDP |
Test Direct Pharmacy | 1234567 |
Test WOL Registered Pharmacy | 7654321 |
Test WOL Pending Pharmacy | TestWOLPendingPharmacy |
Test WOL Fax Alert Failure Pharmacy | TestWOLFaxAlertFailurePharmacy |
Test Transmission Failure | TestTransmissionFailure |
Test ERx Rejected By Pharmacy | TestERxRejectedByPharmacy |
PHP Sample code for sending a message
try { $message = ''; echo $message; $soapclient = new SoapClient('https://cert.wenoexchange.com/wenox/service.asmx'); $param=array('inputString'=>$message); $response =$soapclient->wenoswitch($param); var_dump($response); $array = json_decode(json_encode($response), true); print_r($array); foreach($array as $item) { var_dump($item); } } catch(Exception $e) { echo $e->getMessage(); }
C# Code to Send
using System; using System.IO; using System.Net; using System.Text; using System.Net; namespace soapclient { public class Program { // WENOOnlinePostRx request static void Main(string[] args) { // convert rx to base 64 string rx = Convert.ToBase64String( Encoding.UTF8.GetBytes(File.ReadAllText(@"C:\Users\fed\Source\Workspaces\SoapClient\SoapClient\rx.xml", Encoding.UTF8))); SendMessageToWeno(rx); // send base 64 rx to weno - be sure rx tags contain valid info like unique message ID and credentials Console.ReadLine(); } public static void SendMessageToWeno(string message) { // create WENO Online web request HttpWebRequest request = (HttpWebRequest)WebRequest.Create(@"https://cert.wenoexchange.com/wenox/service.asmx"); request.ContentType = "application/soap+xml"; request.Method = "POST"; request.KeepAlive = false; // soap message calling WENOOnlinePostRx Op using message as inputString parameter // - set WenoOnlineLocationID and SentTime // - encode inputString value to replace <> with < and > to avoid XSS attack warnings string payload = @"<?xml version='1.0' encoding='utf-8'?> <soap12:Envelope xmlns:xsi='http://www.w3.org/2001/XMLSchema-instance' xmlns:xsd='http://www.w3.org/2001/XMLSchema' xmlns:soap12='http://www.w3.org/2003/05/soap-envelope'> <soap12:Body> <WENOOnlinePostRx xmlns='http://tempuri.org/'> <inputString> " + WebUtility.HtmlEncode(string.Format(@" <Message xmlns='https://wenoexchange.com/schema/POSTRX'> <Header> <SentTime>2019-09-09T18:16:54.68</SentTime> </Header> <Body> <PostRxMsg> <WenoOnlineLocationID>L1255</WenoOnlineLocationID> <ValidRxMsg> {0} </ValidRxMsg> </PostRxMsg> </Body> </Message>", message)) + @" </inputString> </WENOOnlinePostRx> </soap12:Body> </soap12:Envelope> "; byte[] byteArray = Encoding.UTF8.GetBytes(payload); request.ContentLength = byteArray.Length; Stream requestStream = request.GetRequestStream(); requestStream.Write(byteArray, 0, byteArray.Length); requestStream.Close(); HttpWebResponse response = null; try { response = (HttpWebResponse)request.GetResponse(); } catch (WebException ex) { response = (HttpWebResponse)ex.Response; } Console.WriteLine(string.Format("HTTP/{0} {1} {2}", response.ProtocolVersion, response.StatusCode, response.StatusDescription)); Stream responseStream = response.GetResponseStream(); StreamReader reader = new StreamReader(responseStream); Console.WriteLine(WebUtility.HtmlDecode(reader.ReadToEnd())); reader.Close(); requestStream.Close(); responseStream.Close(); response.Close(); } } }
Sample Response when sending PostRx
<Message xmlns="https://wenoexchange.com/schema/POSTRX"> <Header> <SentTime>2019-09-04T05:59:34</SentTime> </Header> <Body> <IFrameURL>https://test.wenoexchange.com/en/newrx/reviewrx?token=d1a11f6c92ec4d4d85563d681ca63d4a</IFrameURL> </Body> </Message>
When you flag an eRx message for Return Receipt, you will receive the VERIFY message (1 or more depending on the scenario). It is appropriate to show these to the prescriber.
VERIFY messages will be sent to your endpoint only if the return receipt is flagged on any eRx message.
Verify Code | Description IF … | Then populate the text field of the Verify Message with this text (data type = an1…70) |
---|---|---|
010 | Text 1 scenario | Sent eRx to recipient software system |
010 | Text 2 scenario | Sent eRx to recipient’s confirmed WENO Online account |
010 | Text 3 scenario | Sent eRx and faxed instructions on how to legally retrieve |
010 | Text 4 scenario | Sent eRx but fax alert to recipient failed. WENO will resolve. (NOTE: when you get a Verify message with the description of Text 4, you will get an additional Verify message with the description of Text 3 when WENO resolves it.) |
NCPDP ERROR Message Logic
The Sending Software will always receive ERROR messages when errors occur. This is how they messages are structured and coded so you can understand how to correct them.
Errors will always be sent to your endpoint.
Error Code | What it means |
---|---|
600 | Communication problems – try again later |
601 | Receiver unable to process |
602 | Receiver system error |
700 | Configuration error |
900 | Transaction rejected |
Error “Description Code” can show up to 10 of these in any Error message received. However, the field is not required in the Error message. | |
---|---|
008 | Request timed out before response could be received |
130 | COO Cardholder last name is invalid |
134 | Sending a quantity of 0 is invalid for this pharmacy |
144 | # of refills is invalid |
210 | Unable to process transaction – please resubmit |
220 | Transaction is a duplicate |
500 | XML syntax error – Parser error (NOTE: XPATH of the element in question must accompany in the Description field of this Error so they can isolate and resolve). |
4000 | Intermediary is unable to deliver transaction to the recipient. |
4010 | Intermediary is unable to process response from recipient. |
4020 | Intermediary system error |
4030 | Sender not allowed to send this transaction type |
4040 | Receiver does not support this transaction type |
1000 | Unable to identify based on information submitted (NOTE: XPath of the element in question should be included in the Description field of the Error so they can isolate and resolve). |
2000 | Data format is valid for the element – but content is invalid for the situation (NOTE: XPath of the element in question should be included in the Description field of the Error so they can isolate and resolve) |
3000 | Does not follow the NCPDP standard of implementation guide rules (NOTE: XPath of the element(s) in question should be included in the Description field of this Error so they can isolate and resolve.) |
Error Description (data type = an) this is also not required but used to provide XPATH of the elements in question and also used internally by WENO when we may discover on WENO Online that a pharmacy has rejected the message (most likely unlawfully) | |
---|---|
WENO’s internal Text#W1 | Pharmacy has rejected this transaction. This rejection could be for reasons not allowed by ePrescribing mandates. Contact WENO to report this pharmacy to the DEA or their state board. |
Sample XPATH issue | WENO will provide the Name of the Element with the error. |
WENO Switch Manage APIs allow to programmatically perform Switch functions within your code.
If you handle a small number of prescribers or pharmacies you can also use the Switch Dashboard and perform these operations manually.
Otherwise we recommend to use the Manage APIs in order to integrate these operation into your EHR.
1. in order to access the Manage APIs you will have to connect to the following Endpoint: https://cert.wenoexchange.com/wenox/service.asmx
2. the operation to call is WenoSwitchManage also available at https://cert.wenoexchange.com/wenox/service.asmx?op=WenoSwitchManage
3. View/Download the Manage APIs Schema to understands parameters and types used by the APIs
XML Messages can be validated using the WENO Message Validator
Operation | Used by | Description | Input | Sample Message |
GetMyPrescribersAndLocations | EHR | Gets Prescriber and Location List | view | |
AddLocation | EHR | Adds a prescriber’s Location | Location Info: a LocatioType instance of the location to add. For the LocationType complex type full specs refer to the Manage APIs Schema | view |
EditLocation | EHR | Edits a prescriber’s Location | LocationID: clinic ID number with “C” prefix (for example C12345) PracticeLocationDetails: see LocationType on schema |
view |
DeleteLocation | EHR | Deletes a prescriber’s Location | LocationID: clinic ID “C” prefix (for example C12345). This deletes an existing location. It will also remove the location from all assigned prescribers. If a prescriber is only assigned to the location being deleted, it will cause an error in Script messages because the prescriber location will not be available |
view |
AddPrescriber | EHR | Adds a Prescriber | Prescriber: see Veterinarian and NonVeterinarian types on schema | view |
EditPrescriber | EHR | Edits a Prescriber | PrescriberID: use the Prescriber ID with “D” prefix (for example D12345) Prescriber: see Veterinarian and NonVeterinarian types on schema |
view |
DeletePrescriber | EHR | Deletes a Prescriber | PrescriberID: use the Prescriber ID “D” prefix (for example D12345) | view |
GetMyRegisteredPharmacies | PTV | Gets Registered Pharmacy List | view | |
AddPharmacy | PTV | Adds a Pharmacy | Pharmacy: see PharmacyType on schema | view |
EditPharmacy | PTV | Edits a Pharmacy | PharmacyID: see RequiredUniqueID on schema for US and NON-US pharmacies UpdatePharmacyDetails: see PharmacyType on schema |
view |
DeletePharmacy | PTV | Deletes a Pharmacy | PharmacyId: see RequiredUniqueID on schema for US and NON-US pharmacies | view |
If your EHR has selected free ePrescribing transactions (rather than be charged $0.05/ea) then this is your assigned BSURERXCard info:
- ProcessorIdentificationNumber – Use your WENO Partner ID
- IIN Number – 610280
- CardholderID: use patient 10 digits phone
- GroupID – DFST23
Sample with 2 loops – one is required for the BSURE RX Savings Card and one for any other insurances. It always good to give this info to pharmacies.
<NewRx> <ReturnReceipt>001</ReturnReceipt> <AllergyOrAdverseEvent> <NoKnownAllergies>Y</NoKnownAllergies> </AllergyOrAdverseEvent> <BenefitsCoordination> <PayerIdentification> <ProcessorIdentificationNumber>Use Partner ID, for example 234</ProcessorIdentificationNumber> <IINNumber>610280</IINNumber> </PayerIdentification> <CardholderID>Patient Phone or Zip Code</CardholderID> <GroupID>DFST23</GroupID> <PayerType>L</PayerType> </BenefitsCoordination> <BenefitsCoordination> <PayerIdentification> <ProcessorIdentificationNumber>CRRT</ProcessorIdentificationNumber> <IINNumber>9876784</IINNumber> </PayerIdentification> <CardholderID>2342344</CardholderID> <GroupID>UV3423</GroupID> <PayerType>H</PayerType> </BenefitsCoordination> </NewRx>
PayerType Codes Explained
- A – Medicare Part A
- B – Medicare Part B
- C – Medicare Part C
- D – Medicare Part D
- E – Medicaid
- F – Managed Care
- G – Hospice
- H – Commercial
- J – Private Pay
- K – Worker’s Comp
- L – Discount Program (This is used for our BSURE Rx Savings Card)
- M – Coupon
- N – Voucher
- P – Military/VA
- ZY – Other
- ZZ – Unknown
Check if testing is complete:
- Sent valid NewRx successfully
- DaysSupply is present
- Written DATE/Time is present and in UTC – must represent prescriber’s electronic signature date/time of NewRx message
- Sent DATE/Time is in UTC
- Prescriber Suffix field is present
- Place of Service field in prescriber and/or supervisor segments is present. For Place of Service list click here
- Sent midlevel prescriber NewRx with Supervisor field present (Supervisor has same fields as prescriber including Suffix present)
- Sent NewRx with Diagnosis at least once
- Sent NewRx with EPCS to show error if DEA audit is not found and to process if DEA audit is on file
- Benefit Coordination Section completed correctly
- Sent NewRx with Effective Date used correctly
- Sent NewRx for patient under 18 with height and weight present
- Practiced failed transmission correctly (Endpoint is working)
- Practiced refusedRX correctly
- Use of D & C qualifier in FROM field (D is mandatory). This is a 2 part ID which identifies the prescriber (D value) and the location (C value) separated by a colon like for example D1234:C987098. WENO will return the WENO PrescriberID after you successfully register the prescriber either in your dashboard or calling the Manage APIs.
- Use of Manage APIs without issues (if your EHR will use the manual process you can skip this)
- Practiced Add Pharmacy that was not on WENO Directory
When all testing is complete, contact your certification manager to move to live.